list
更新时间:
列表视图容器,仅支持<list-item>
子组件,支持通用属性,支持通用样式
属性
名称 | 类型 | 默认值 | 必填 | 描述 |
---|---|---|---|---|
type | normal|fisheye|grid | normal | 否 | 设置列表的的布局方式,默认为普通的 list 布局;fisheye 是类似鱼眼镜头效果布局;grid 是网格布局。该属性不可动态变更 |
scrollbar | <boolean> |
false | 否 | 是否启用滚动条 |
title | <boolean> |
false | 否 | 值为 true <list-item> 的第一个元素将会作为 list 的标题,标题位置固定在 list 开头,向上滑动时标题会渐渐消失(透明度逐渐变为完全透明) |
circular | <boolean> |
false | 否 | 是否循环展示 <list-item>, 值为 true 时部分滚动事件不可用。 |
alignmentnum | <number> |
3 | 否 | 鱼眼 list 一屏幕 <list-item> 对齐数量,type 为 fisheye 时生效,设置范围:3-7,超出范围则自动设置为默认值 |
bounces | <boolean> |
true | 否 | 是否启用回弹 |
样式
名称 | 类型 | 默认值 | 必填 | 描述 |
---|---|---|---|---|
flex-direction | column | row | column | 否 | - |
columns | <number> |
1 | 否 | list 显示列数 |
事件
名称 | 参数 | 描述 |
---|---|---|
scrollbottom | - | 列表滑动到底部 |
scrolltop | - | 列表滑到到顶部 |
scrollindex | {first, last} | 返回 list 可视范围的索引范围。first:可视范围第一个 item 的索引;last:可视范围最后一个 item 的索引。 |
方法
名称 | 参数 | 描述 |
---|---|---|
scrollTo | Object | list 滚动到指定 item 位置 |
scrollTo 的参数说明:
名称 | 类型 | 是否必选 | 默认值 | 备注 |
---|---|---|---|---|
index | number | 是 | 无 | list 滚动的目标 item 位置 |
behavior | smooth|instant|auto | 否 | auto | 是否平滑滑动,支持参数 smooth (平滑滚动),instant (瞬间滚动),默认值 auto,效果等同于 instant |
list组件示例
列表吸顶
<template>
<div class="wrapper">
<list class="list" bounces="false">
<list-item type="ceiling">
<text class="title">{{ title }}</text>
</list-item>
<list-item type="item" for="{{ count }}">
<text class="text">{{ $item }}</text>
</list-item>
</list>
<text class="ceiling title">{{ title }}</text>
</div>
</template>
<script>
export default {
data() {
return {
count: Array.from({ length: 20 }, (v, i) => i),
title: 'list ceiling'
}
}
}
</script>
<style>
.wrapper {
flex-direction: column;
}
.title,
.text {
height: 180px;
width: 480px;
text-align: center;
font-size: 50px;
background-color: #203a43;
color: #ffffff;
border-bottom: 1px solid #2c5364;
}
.title {
background-color: #0f2027;
}
.ceiling {
position: fixed;
top: 0;
left: 0;
pointer-events: none;
}
</style>
复制代码
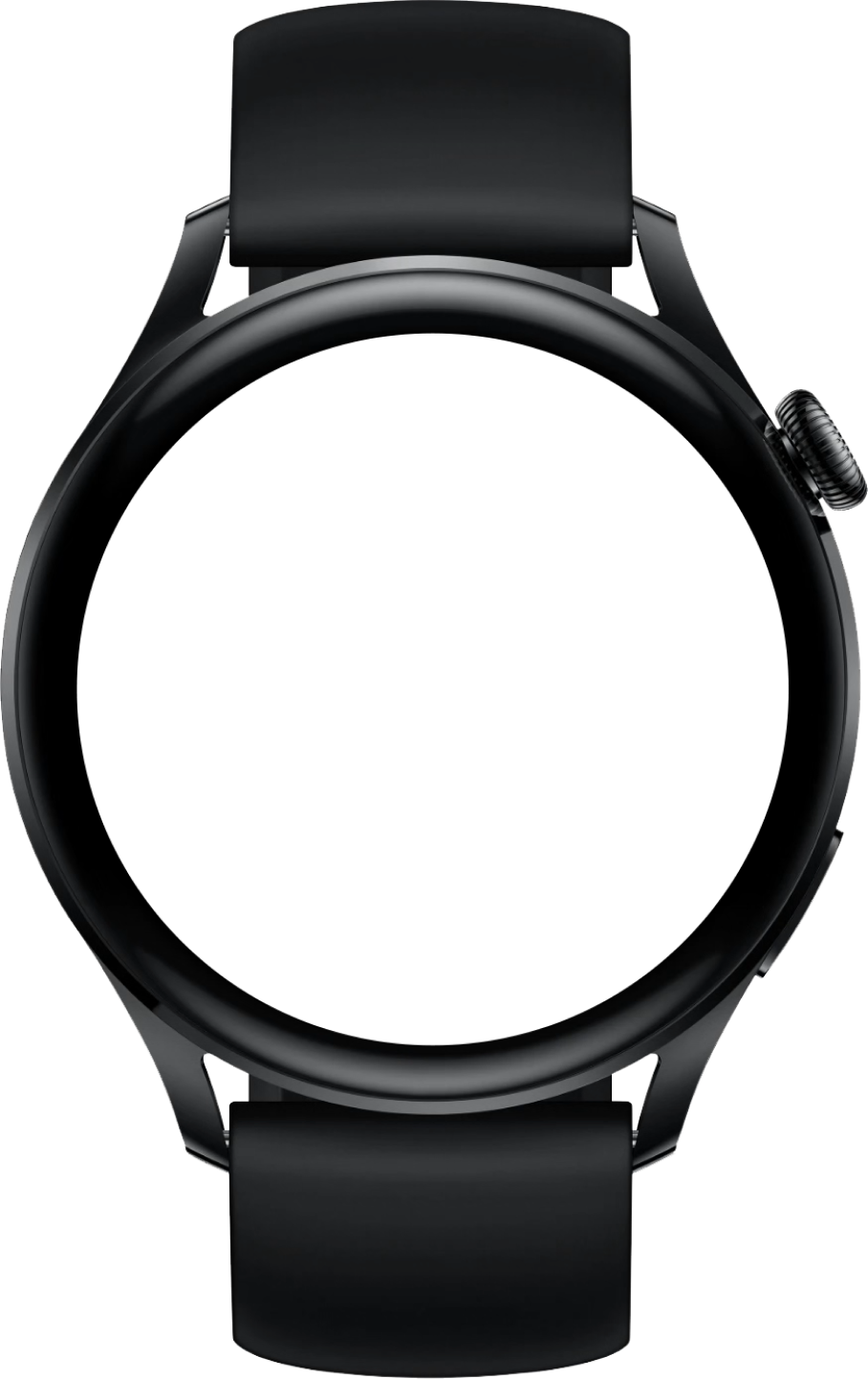
鱼眼列表
<template>
<list class="wrapper" type="fisheye" alignmentnum="{{ alignmentnum }}">
<list-item type="item" class="list-item" for="{{ count }}">
<text class="text">{{ $item }}</text>
</list-item>
</list>
</template>
<script>
export default {
data() {
return {
count: Array.from({ length: 20 }, (v, i) => i),
alignmentnum: 4
}
}
}
</script>
<style>
.list-item {
align-items: center;
justify-content: center;
}
.text {
width: 680px;
height: 180px;
font-size: 50px;
text-align: center;
background-color: #e8f3fe;
color: #7dbcfc;
}
</style>
复制代码
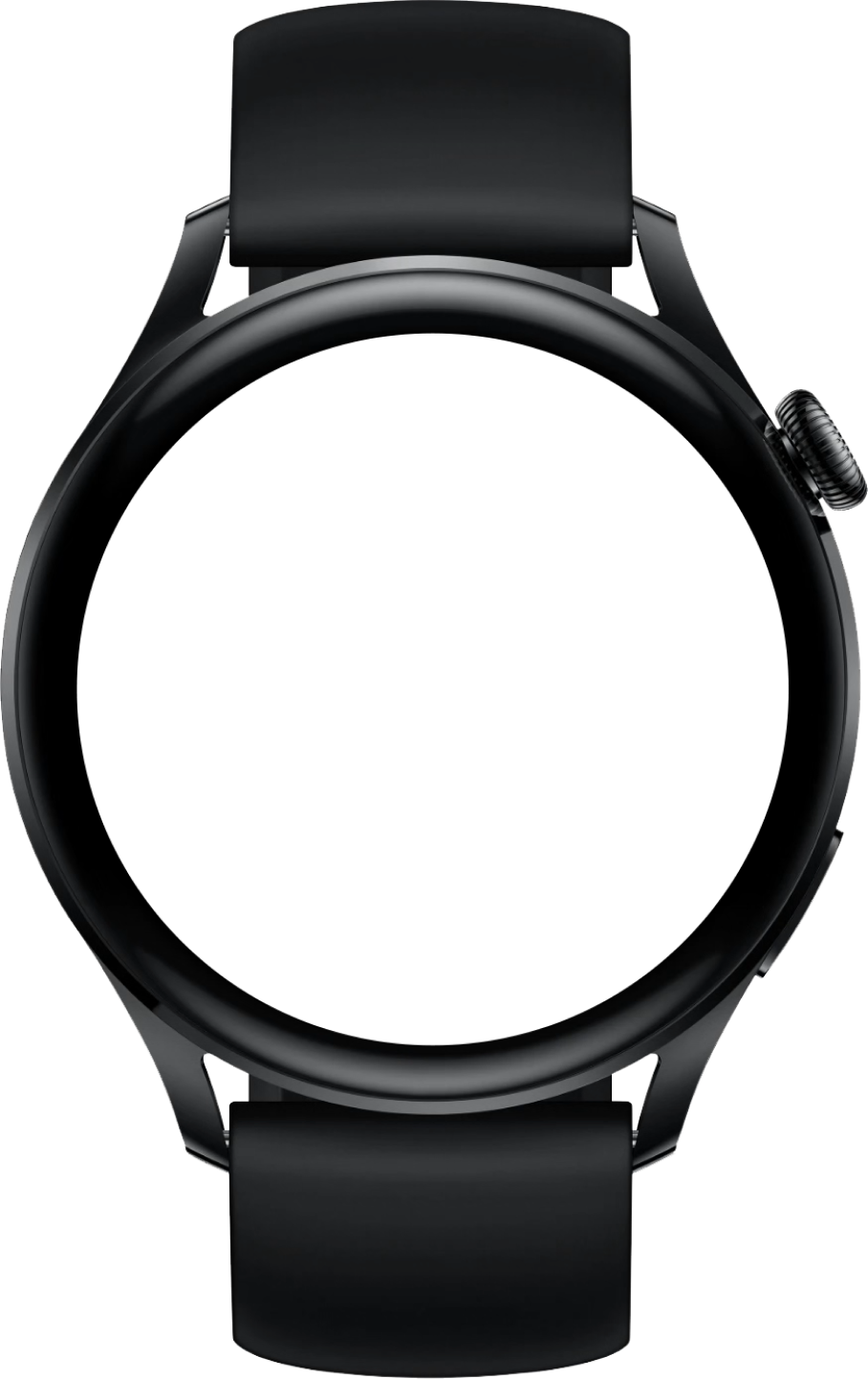
网格列表
<template>
<list class="wrapper" type="grid">
<list-item
type="item"
class="list-item {{ $idx % 2 === 0 ? '' : 'list-odd' }}"
for="{{ count }}"
>
<text class="text">{{ $item }}</text>
</list-item>
</list>
</template>
<script>
export default {
data() {
return {
count: Array.from({ length: 20 }, (v, i) => i)
}
}
}
</script>
<style>
.wrapper {
columns: 3;
}
.list-item {
height: 180px;
align-items: center;
justify-content: center;
background-color: #e8f3fe;
column-span: 1;
}
.text {
font-size: 50px;
text-align: center;
color: #7dbcfc;
}
.list-odd {
background-color: #6a88e6;
color: #ffffff;
}
</style>
复制代码
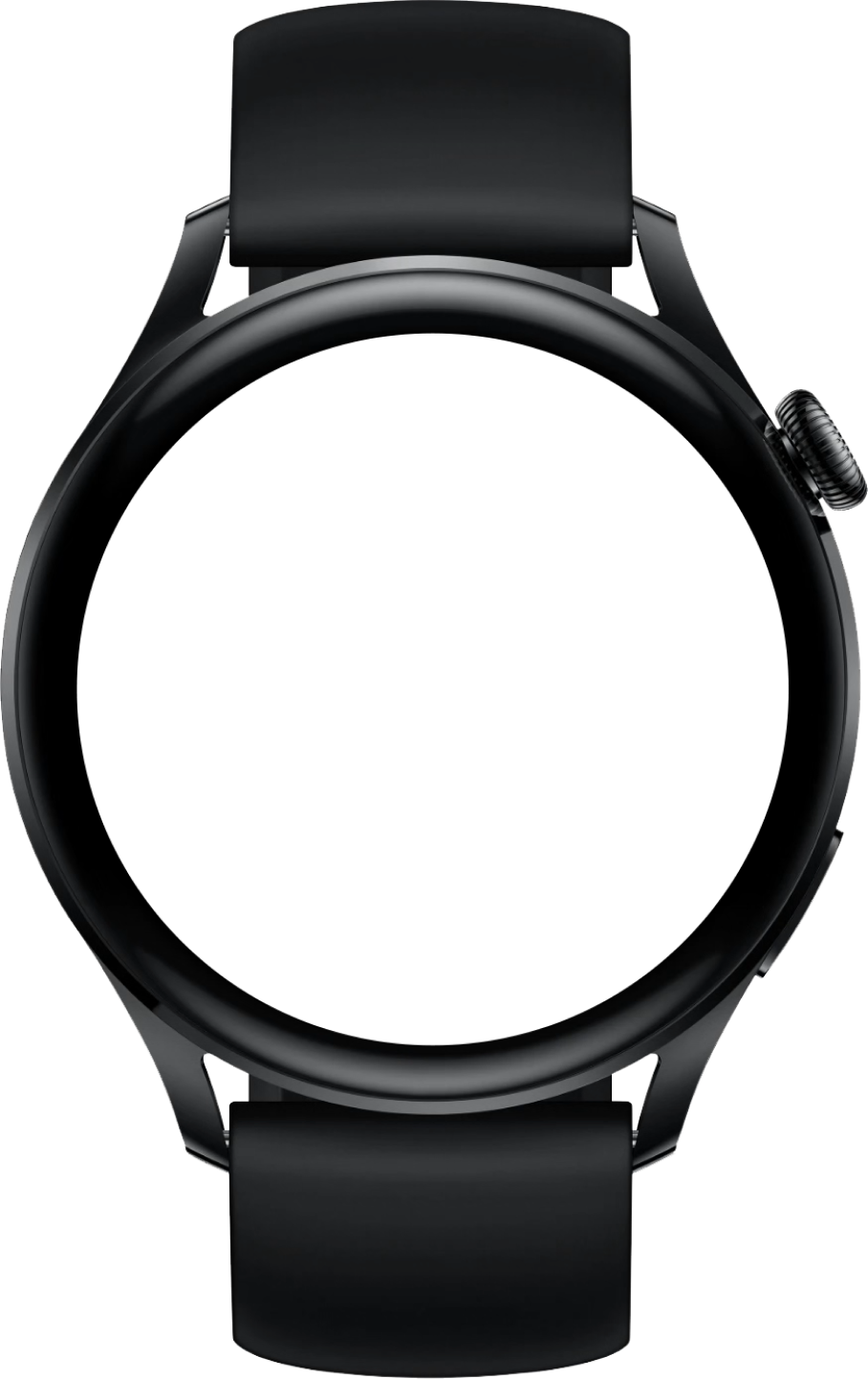
列表加载更多
<template>
<list class="wrapper" onscrollbottom="loadMoreHandler">
<list-item type="item" for="{{ count }}">
<text class="item {{ $idx >= defaultLen ? 'added' : '' }}">{{
$idx
}}</text>
</list-item>
</list>
</template>
<script>
const default_len = 20
export default {
data() {
return {
count: Array.from({ length: default_len }, (v, i) => i),
defaultLen: default_len
}
},
loadMoreHandler() {
setTimeout(() => {
this.count.push(this.count.length)
}, 500)
}
}
</script>
<style>
.wrapper {
background-color: #232526;
}
text {
width: 480px;
height: 180px;
text-align: center;
font-size: 50px;
color: #ffffff;
border-bottom: 1px solid #414345;
}
.added {
background-color: #203a43;
border-color: #2c5364;
}
</style>
复制代码
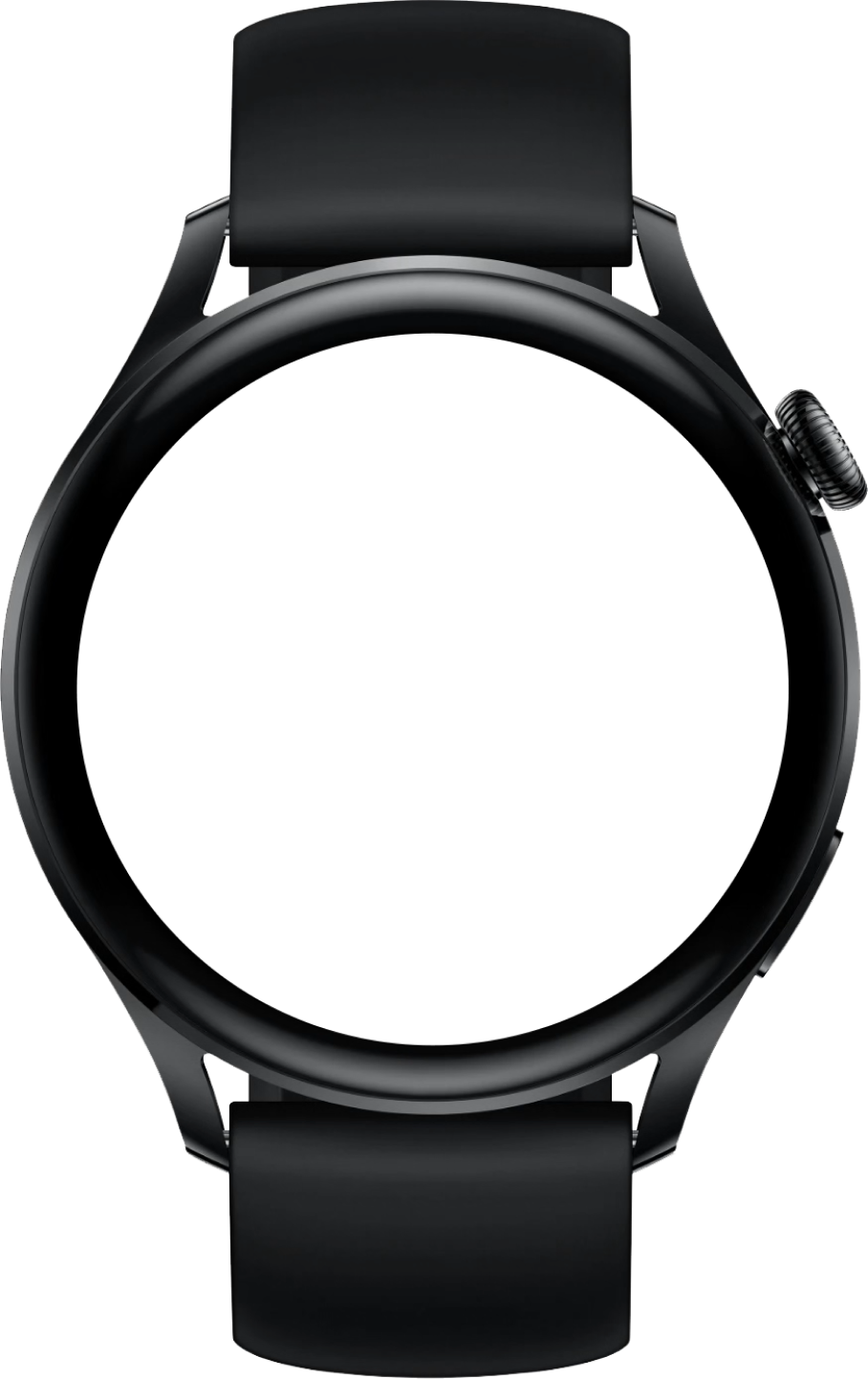
基本用法
<template>
<list class="wrapper">
<list-item type="item" class="list-item" for="{{ count }}">
<text class="text">{{ $item }}</text>
</list-item>
</list>
</template>
<script>
export default {
data() {
return {
count: Array.from({ length: 20 }, (v, i) => i)
}
}
}
</script>
<style>
.text {
width: 480px;
height: 180px;
font-size: 50px;
text-align: center;
color: #ffffff;
border-bottom: 1px solid #414345;
background-color: #232526;
}
</style>
复制代码
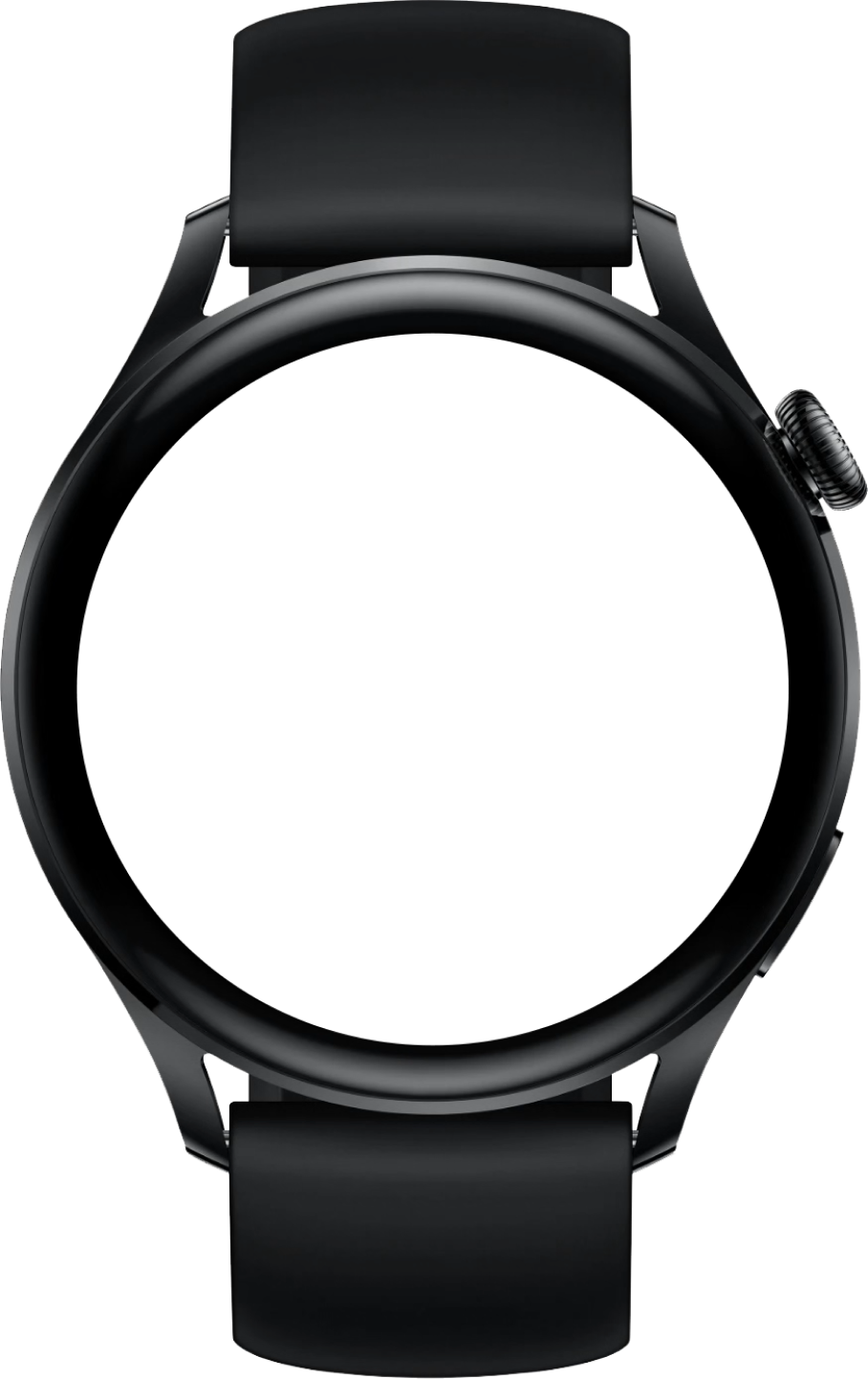